Table.TransformRows Function in Power Query
The Table.TransformRows function transforms the rows from a table using a transform function and return the result as list.
Syntax
Table.TransformRows(table as table, transform as function) as list
Example:
Power Query M
let MyTable = Table.FromRecords( { [CustomerID = 1, Name = "Ashish", Phone = "123-4567"], [CustomerID = 2, Name = "Katrina", Phone = "987-6543"], [CustomerID = 3, Name = "Alia", Phone = "543-7890"], [CustomerID = 4, Name = "Vicky", Phone = "676-8479"], [CustomerID = 5, Name = "Mohini", Phone = "574-8864"], [CustomerID = 6, Name = "Meenakshi", Phone = "574-8864"], [CustomerID = 7, Name = "Esha", Phone = "574-8864"], [CustomerID = 8, Name = "Anjali", Phone = "574-8864"] } ), Return = Table.TransformRows(MyTable, each _) in Return
The output of the above code is shown below:
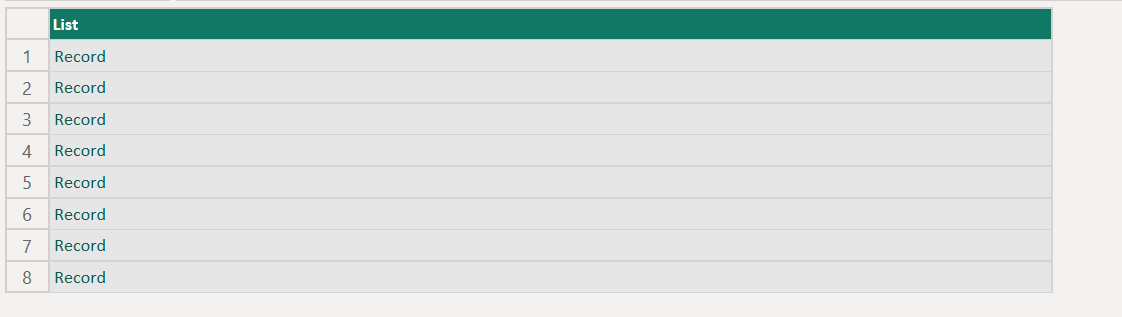