Series in Pandas
A Pandas Series is a one-dimensional labeled array capable of holding data of any type (integer, float, string, Python objects, etc.). It is similar to a column in a spreadsheet or a database table. Each element in a Series has a unique label, called an index, which can be used to access its values.
Features of a Pandas Series: 1. Homogeneous Data: All elements in a Series are of the same type.
2. Index: Each element has an associated index label, which defaults to integers starting from 0.
3. Mutability: The data in a Series can be modified.
Create Pandas Series
Let’s create a Pandas Series.
Syntax pandas.Series(data=None, index=None)
Example: Creating a Series from a list.
Python
data = [10, 20, 30, 40, 50] series = pd.Series(data) print(series)
The output of the above code is shown below:
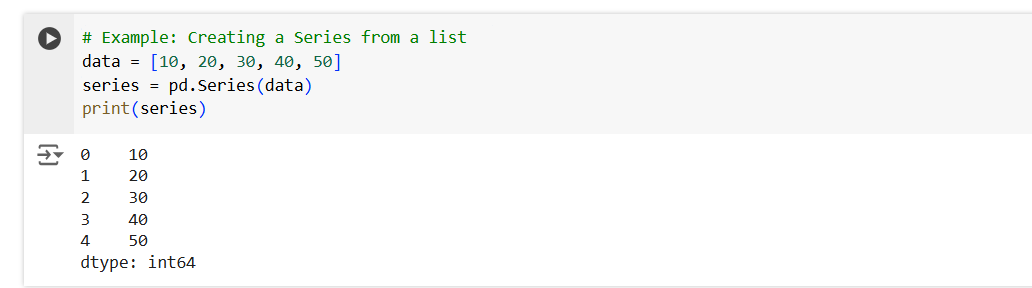
We can access elements using the index.
Python
The output of the above code is 10.
Example: Creating a Series with custom index.
Python
data = [100, 200, 300] index = ['a', 'b', 'c'] newseries = pd.Series(data, index=index) print(newseries)
The output of the above code is shown below:
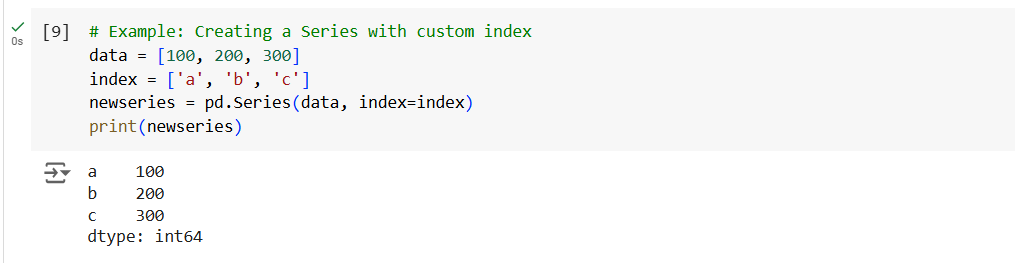
Here, we also we can access the element using the custom index.
Python
print(newseries[‘b’])
The output of the above code is 200.
Series Functions and Properties Here, we are going to discuss some common functions and properties of the Series in Pandas.
1. Series.sum() The Series.sum() function is used to return the sum of the values of the series.
As we have already created newseries, lets get the sum of the values of the newseries.
Python
The output of the above code is 600.
2. Series.min() The Series.min() function is used to return the minimum value of the series.
Python
The output of the above code is 100.
3. Series.max() The Series.max() function is used to return the maximum value of the series.
Python
The output of the above code is 300.
4. Series.product() The Series.product() function is used to return the product of the value of the series.
Python
The output of the above code is 6000000.
5. Series.size The Series.size property in series used to return the number of the items in the series.
Python
The output of the above code is 3.
6. Series.empty The Series.empty property in series used to check whether the series is empty or not and it returned the boolean value. It returns True if Series is entirely empty (no items), otherwise False.
Python
The output of the above code is False.
7. Series.pop The Series.pop property in series used to drop the item from the series at the given index and returned that item value which is dropped.
Python
poppeditem=newseries.pop('b') poppeditem
The output of the above code is 200.
Now when we check the newseries variable, it is also updated, as shown in the image below.
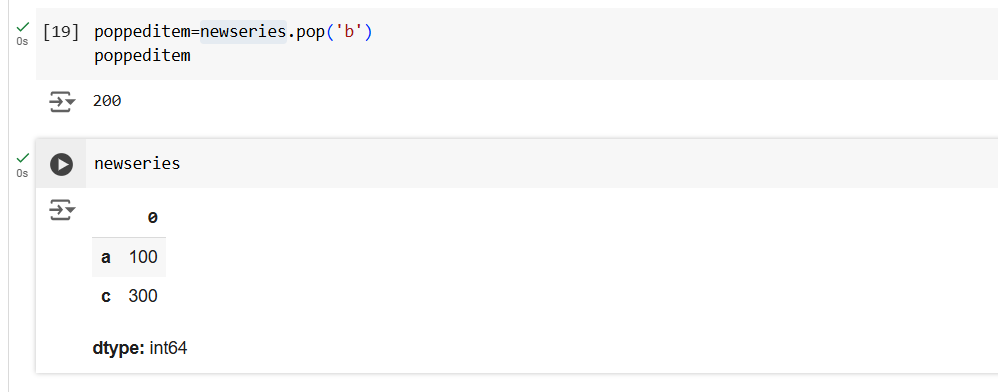
pandas.Series.str.lower The series.str.lower() function is used to converts all characters to lowercase.
Example: Create a series.
Python
data = ["Ashish", "Katrina", "Alia", "Esha", "Anushka"] newseries = pd.Series(data) print(newseries)
The below command is used to lowercase the characters in the series.
Python
The output of the about code is shown in the image below:
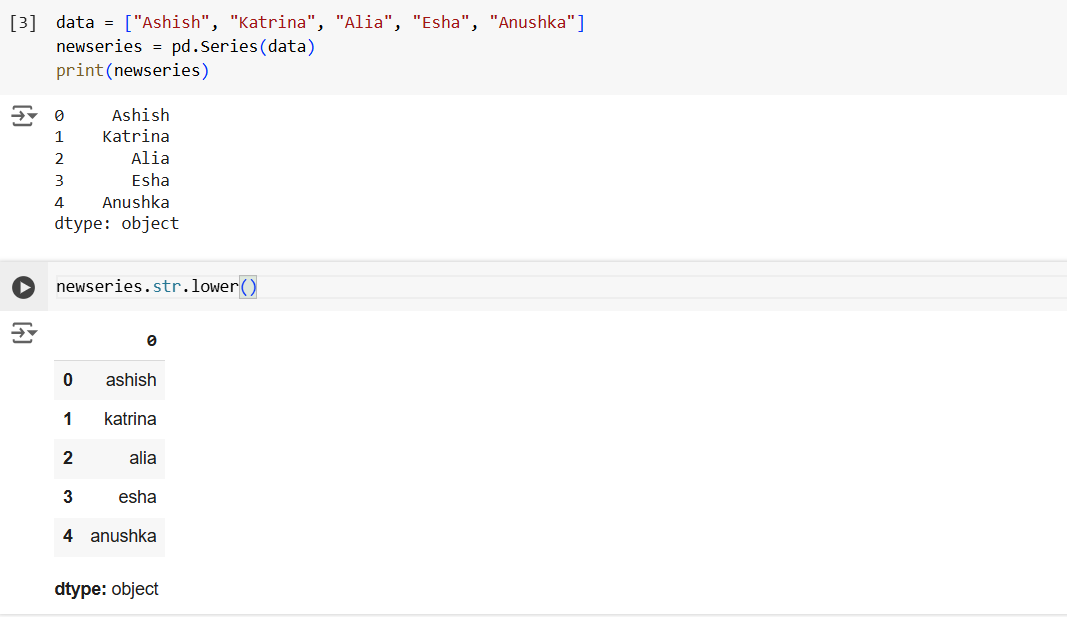
pandas.Series.str.upper The series.str.upper() function is used to converts all characters to uppercase.
Example: Create a series.
Python
data = ["Ashish", "Katrina", "Alia", "Esha", "Anushka"] newseries = pd.Series(data) print(newseries)
The below command is used to uppercase the characters in the series.
Python
The output of the about code is shown in the image below:
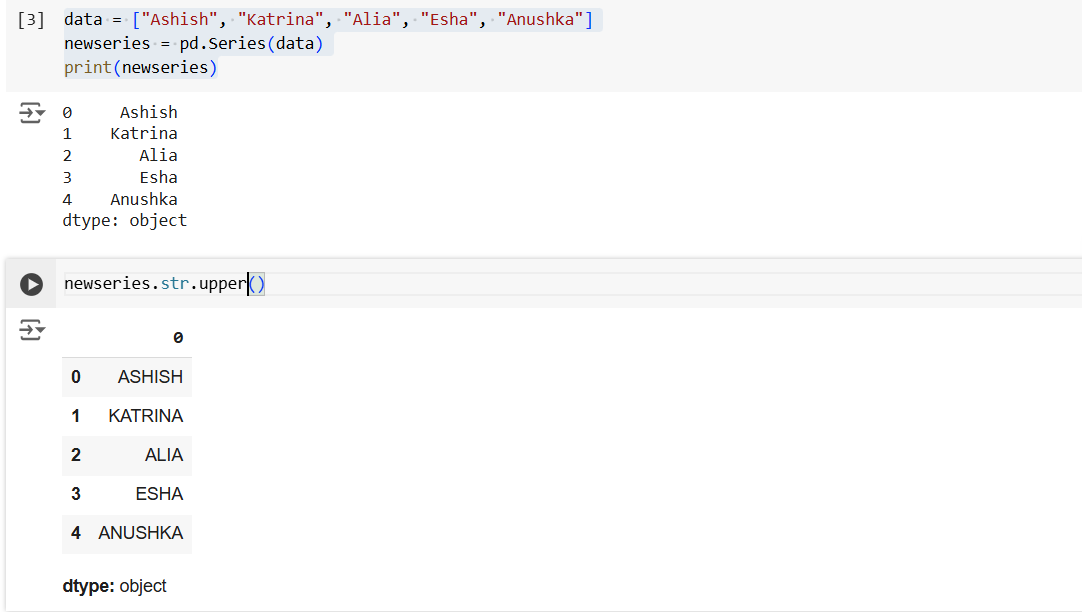
pandas.Series.str.len The series.str.len() function is used to get the length of the characters in the item value.
Example: Create a series.
Python
data = ["Ashish", "Katrina", "Alia", "Esha", "Anushka"] newseries = pd.Series(data) print(newseries)
The below command is used to get the length of the characters in the series.
Python
The output of the about code is shown in the image below:
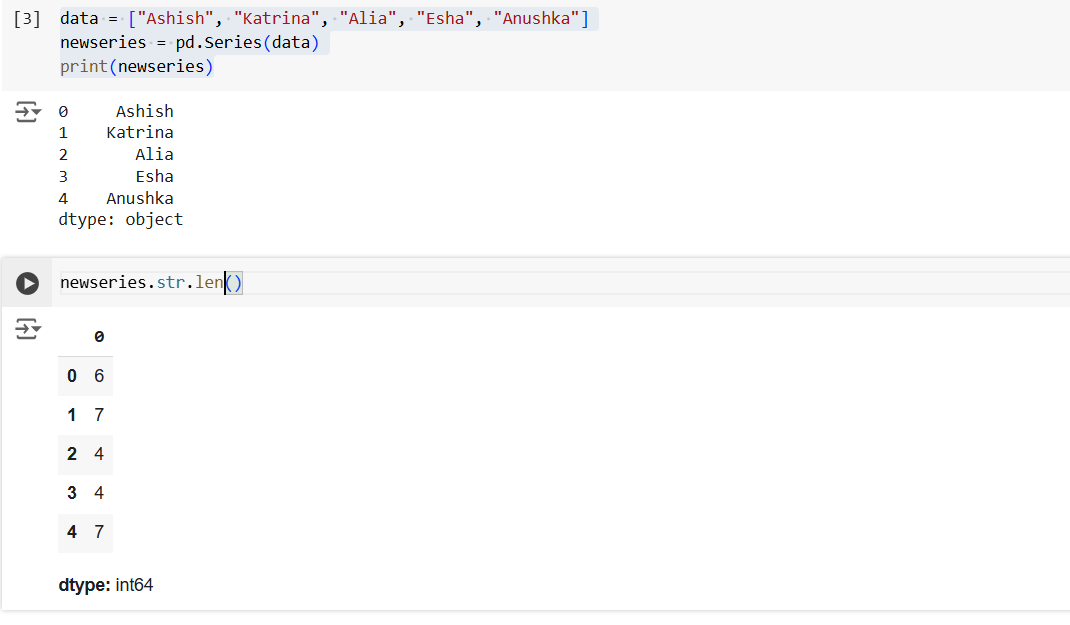