Pandas- An Introduction to Pandas
Pandas is a data analysis library built on top of the Python programming language.
Install Pandas The pandas can be installed via pip from PyPI using the command pip install pandas.
Note: • To upgrade the already installed pandas’ version we can use the command pip install pandas --upgrade.
• To install the specific version of pandas we can use the command pip install pandas==version_number. For example, pip install pandas==2.2.2
• To uninstall the already installed pandas we can use the command pip uninstall pandas.
To start using pandas we first need to import the pandas package by using the command import pandas as pd.
Parameters and Arguments The definition of Parameters and Arguments are shown below:
- Parameters: Variables defined in a function or method's signature. They indicate what values you can pass when calling the function.
- Arguments: Actual values passed to these parameters when you call the function.
Example:
Python
import pandas as pd num_data = [100, 200, 300, 400, 500] my_index = ['a', 'b', 'c', 'd', 'e'] # Arguments will be passed to parameters sequentially pd.Series(num_data, my_index)
The output of the above code is shown below:
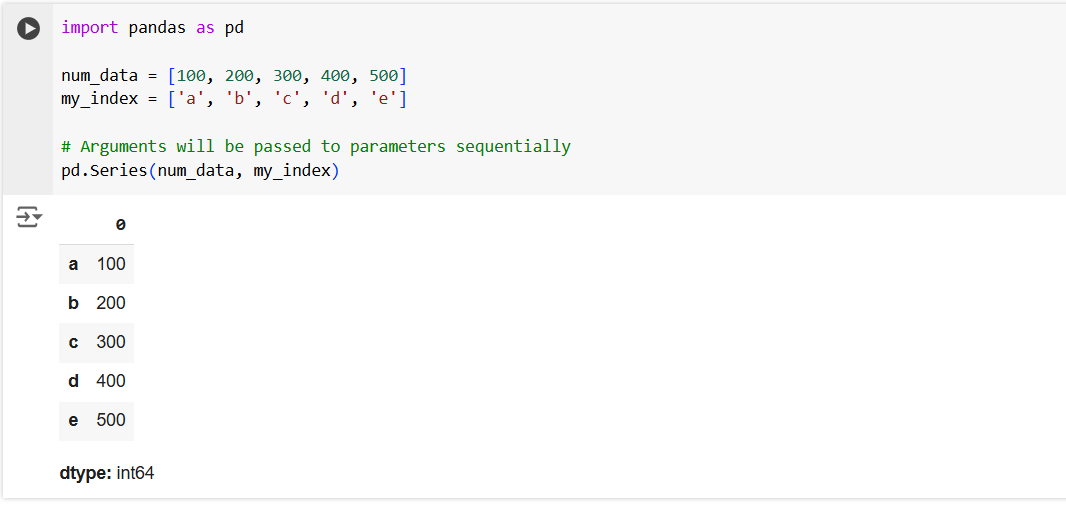
Python
pd.Series(my_index, num_data)
The output of the above code is shown below:
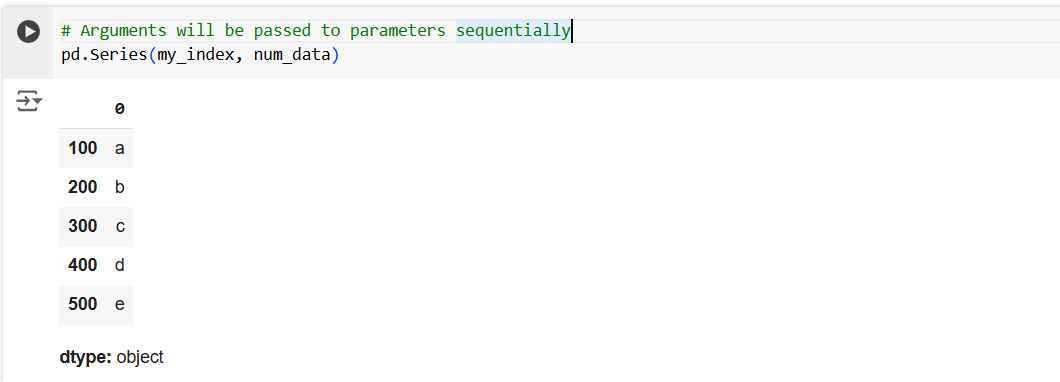
Python
# Use keywords to match parameters and arguments pd.Series(index = my_index, data = num_data) pd.Series(index = my_index, data = num_data)
Both produces the same output. As shown in the image below:
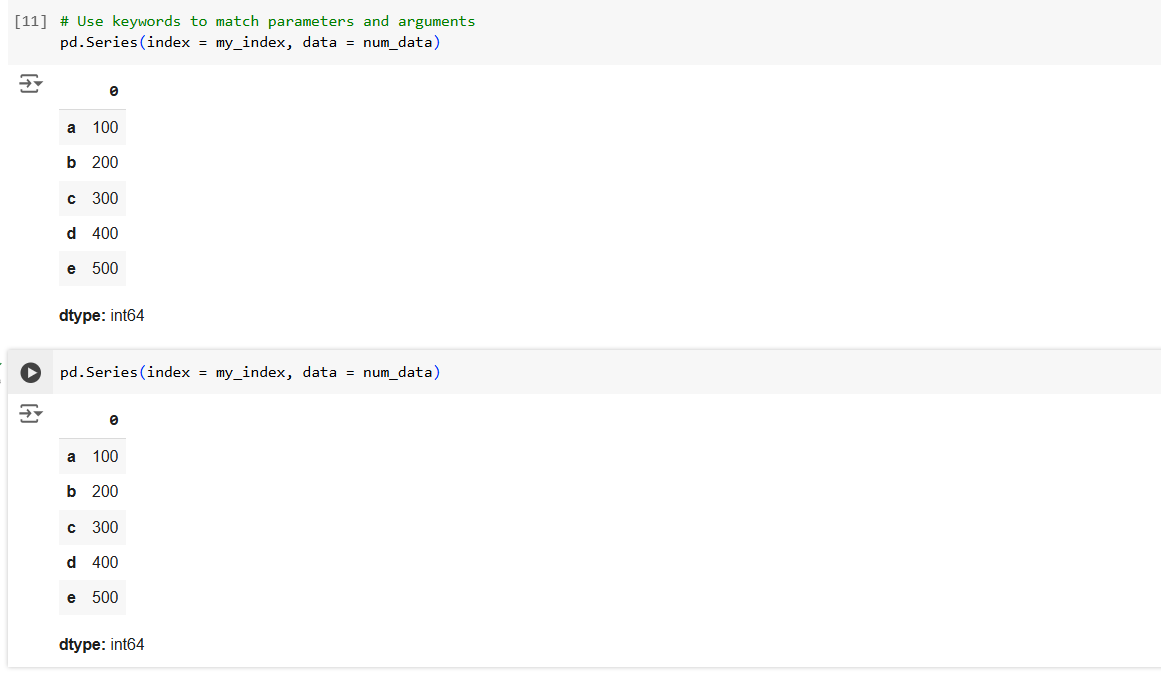
Python
# Some parameters are sequential, and some are keyword arguments pd.Series(num_data, index= my_index)
The output of the above code is shown below:
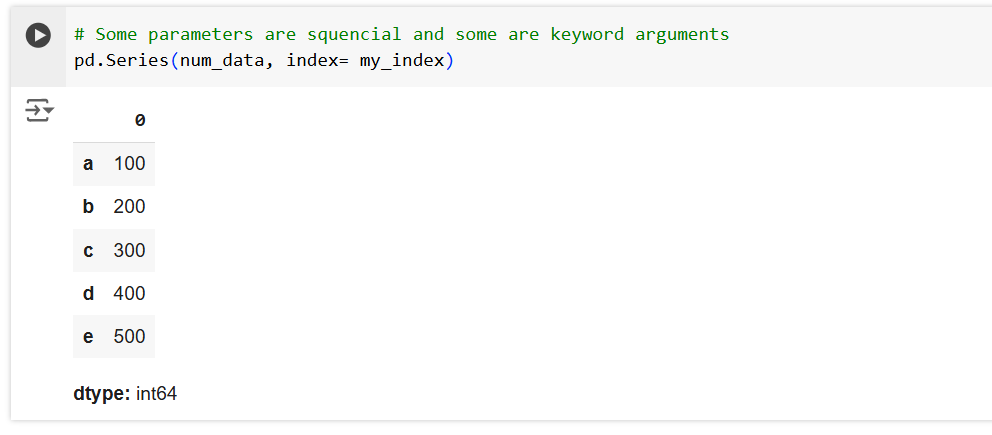