Read Data from CSV file in Pandas
We can use the read_csv function to read the data from the csv file and converts it into a DataFrame. To read the data from the csv file in pandas using the below syntax:
Syntax a) pd.read_csv(“file_name.csv”)
b) pd.read_csv(“file_name.csv”, index_col=None)
c) pd.read_csv(“file_name.csv”, skip_blank_lines=False)
The skip_blank_lines parameter specifies whether the blank rows should be NaN values in the output or just skip those blank rows in the output. The default value for the parameter is True. It means by default it skips the blank rows.
Note: In this exercise, we are using the datasource data.csv. You can download the datasource and use for the transformation.
Example: Here, we are reading the file named data.csv and then show the dataframe in the output.
Python
#Read the data from the data.csv file and create a DataFrame # vardata="data.csv" # mydata=pd.read_csv(vardata) mydata=pd.read_csv("data.csv") mydata
The output of the above code is shown below:
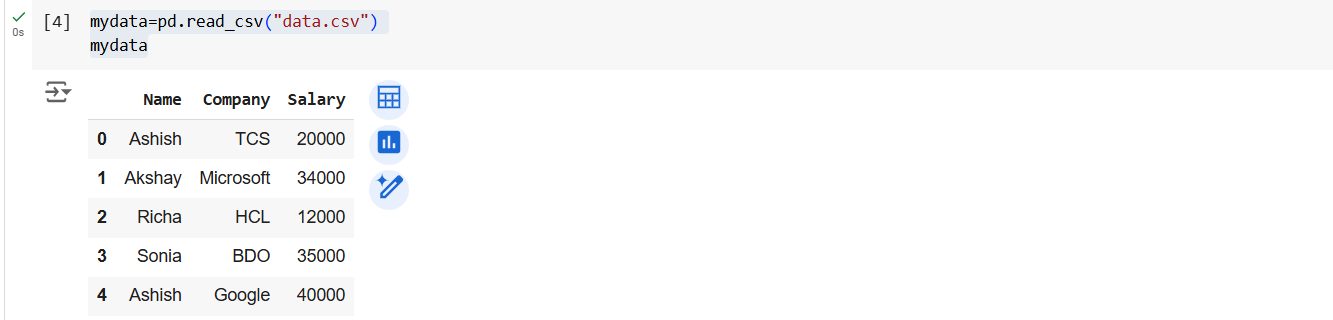
We can use the index_col parameter to specify which column we are going to use as row label.
Python
mydata=pd.read_csv("data.csv", index_col="Name") mydata
The output of the above code is shown below:
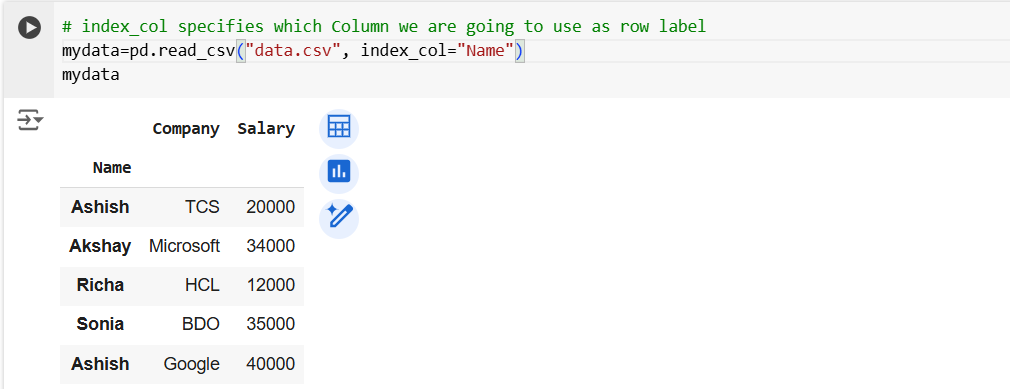
Read Specific Columns Let’s read only two columns to create a DataFrame, we can achieve this by using the usecols parameter, with the list of column names.
Python
mydata=pd.read_csv("data.csv", usecols=["Name", "Company"]) mydata
The output of the above code is shown below:
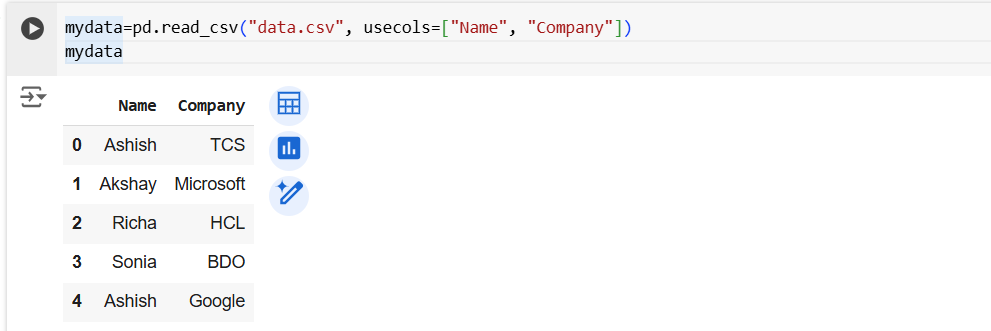