Export DataFrame to CSV File in Pandas
The to_csv method exports a DataFrame to a CSV file. The first argument is the filename. By default, pandas will include the index. Set the index parameter to False to exclude the index. The columns parameter limits the exported columns.
Syntax a) pandas.DataFrame.to_csv(“filepath/filename.csv”)
b) pandas.DataFrame.to_csv(“filepath/filename.csv”, columns, index, header)
• header The header parameter specifies that column names should be available in the output or not. It takes a boolean value, by default it is True. • index The index parameter specifies that row names should be available in the output or not. It takes a boolean value, by default it is True. • columns The columns parameter specifies which column should be available in the output.
Example: Let’s create a DataFrame.
Python
import pandas as pd # Define data mydata = { 'Name': ['Ashish', 'Katrina', 'Alia', 'Ashish', 'Alia'], 'Age': [25, 30, 35, 25, 40], 'City': ['New York', 'Los Angeles', 'Mumbai', 'New York', 'Mumbai'] } # Create a DataFrame df = pd.DataFrame(mydata) print(df)
The output of the above code is shown below:
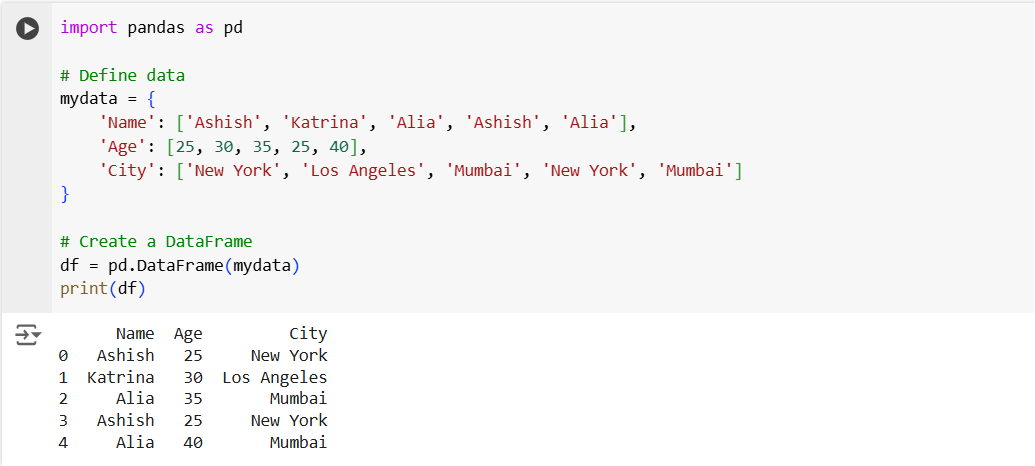
Let’s create a mydata.csv file in the output.
Python
# Export to CSV # This will save the file to the same directory as our notebook is in df.to_csv('mydata.csv')
The output of the above code is shown below:
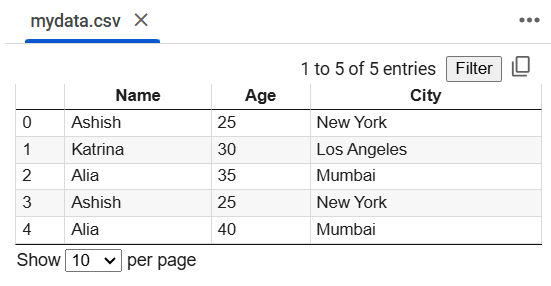
Let’s create a mydata.csv file in the output. But this time we do not want the row name in the output file.
Python
# Export to CSV # we can exclude the index parameter in the output df.to_csv('mydata.csv', index=False)
The output of the above code is shown below:
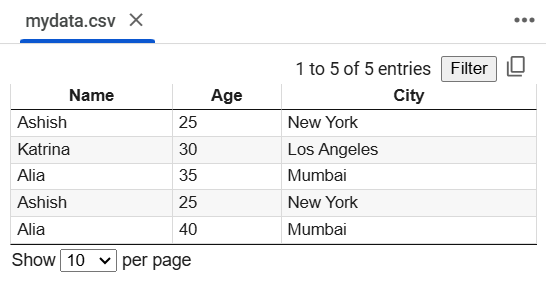
Let’s create again mydata.csv file in the output. But this time we include only the specified columns in the output.
Python
# Export to CSV # We can limit the columns to save in the output df.to_csv('mydata.csv', index=False, columns=['Name', 'Age'])
The output of the above code is shown below:
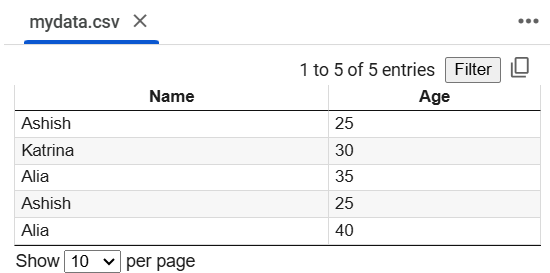
Create a .txt file We not only create the csv file but also by the help of to_csv function we can create the .txt file.
Example: Load the DataFrame.
Python
import pandas as pd # Define data mydata = [['Ashish', 'Katrina', 'Alia', 'Ashish', 'Alia'], [25, 30, 35, 25, 40], ['New York', 'Los Angeles', 'Mumbai', 'New York', 'Mumbai'] ] # Create a DataFrame df = pd.DataFrame(mydata) print(df)
The output of the above code is shown below:
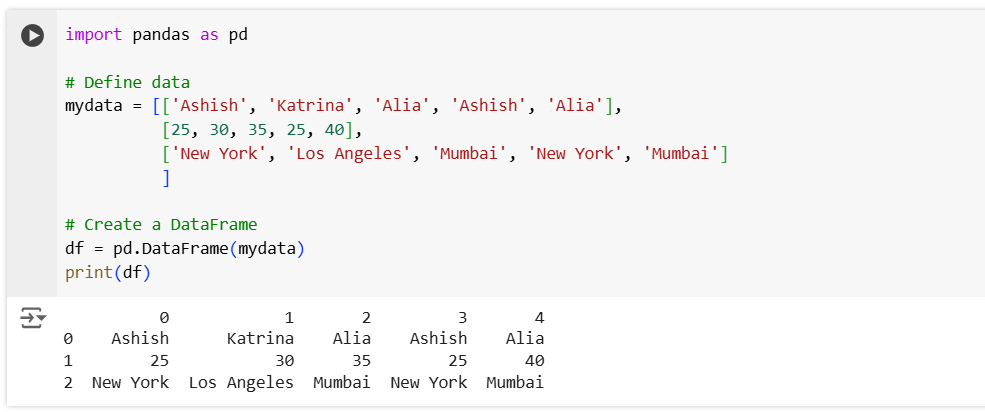
Now, let’s create a .txt file.
Python
# Create a txt file df.to_csv('mydata.txt')
The output of the above code is shown below:
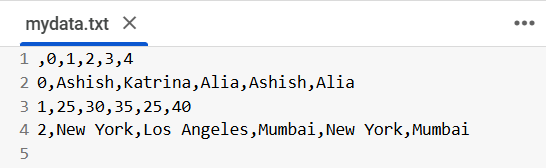
Now in the output we do not want the row labels.
Python
# Create a txt file df.to_csv('mydata.txt', index=False)
The output of the above code is shown below:
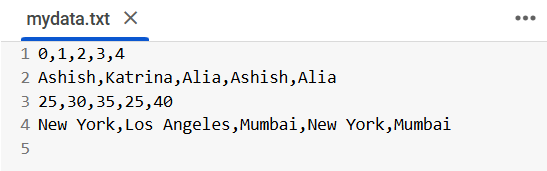
Python
# Create a txt file df.to_csv('mydata.txt', index=False, header=False)
The output of the above code is shown below:
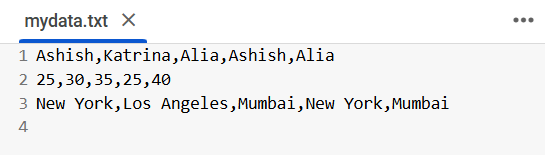