The dropna() method in Pandas
In this exercise, we are using the datasource employeeswithna.csv. You can download the datasource and use for the transformation.
The dropna() method is used to remove the rows with null (NA) values in Series/DataFrame.
Syntax a) pandas.Series.dropna()
It deletes the rows that have null values in the series.
b) pandas.DataFrame.dropna()
It deletes the rows that have any null values in any of the column of the DataFrame.
c) pandas.DataFrame.dropna(how=“any”)
d) pandas.DataFrame.dropna(how=“all”)
The dropna function parameter how has the two values:
• ‘any’: Drop the row, if any column in the row has NA value present, drop that row.
• ‘all’: Drop the row only if all values in the row are NA.
By default, the how parameter has value any.
Example: Load the Dataframe.
Python
import pandas as pd mydata=pd.read_csv("employeeswithna.csv") mydata
The output of the above code is shown below:
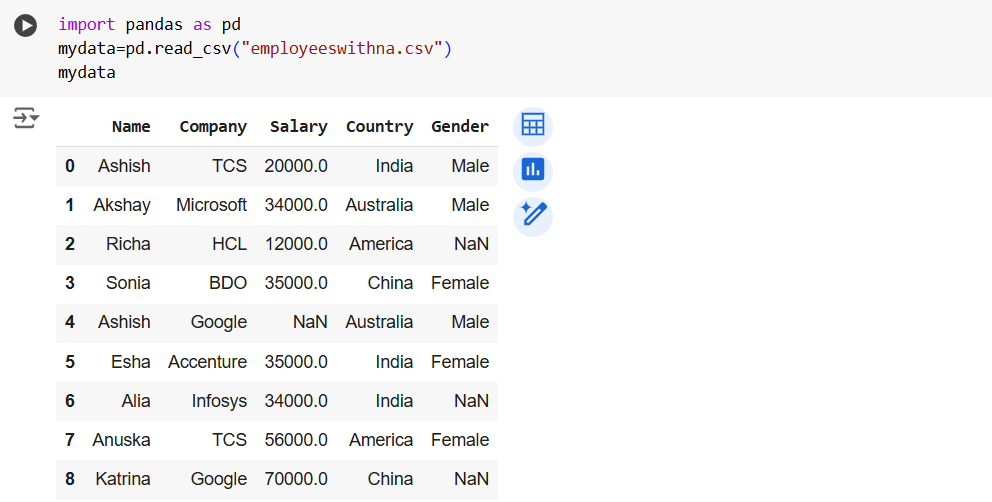
Let’s drop the rows from the DataFrame if any column in the row has value NA.
Python
# Delete rows that have any null values mydata.dropna()
The output of the above code is shown below:
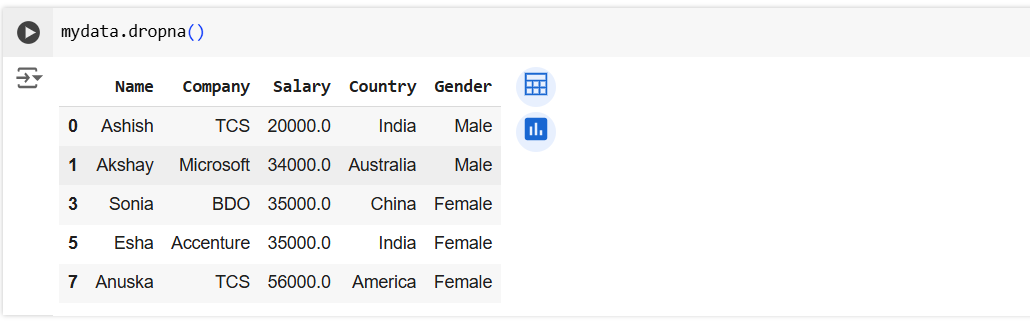
Remove the null values from the Series Let’s extract the series from the DataFrame and execute the dropna() method on that.
Python
# Delete rows that have any null values mydata[“Gender”].dropna()
The output of the above code is shown below:
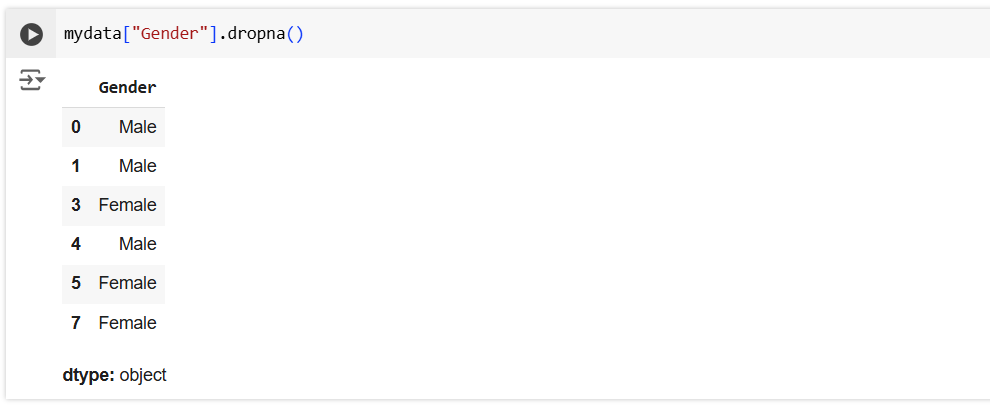