Create a column in Dataframe in Pandas
In this exercise, we are using the datasource employees.csv. You can download the datasource and use for the transformation.
To create a new column, use the [] brackets with the new column name at the left side of the assignment.
Syntax df[“New_Column_Name”] = dataframe-expression
The operations are element-wise, so no need to loop over rows.
Example: Load the DataFrame.
Python
import pandas as pd mydata=pd.read_csv("employees.csv") mydata
The output of the above code is shown below:
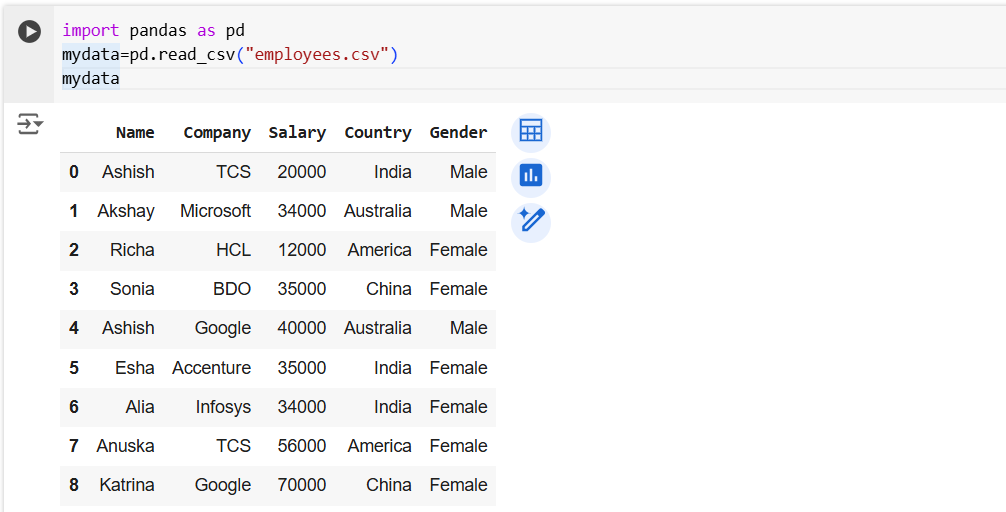
Here we are creating a new column in dataframe with name “NewSalary”. We are adding 1000 in each row of the Salary column.
Python
mydata["NewSalary"] = mydata["Salary"] + 1000 mydata
Or, the above code can be rewritten as:
Python
mydata["NewSalary"] = mydata["Salary"].add(1000) mydata
The output of the above code is shown below:
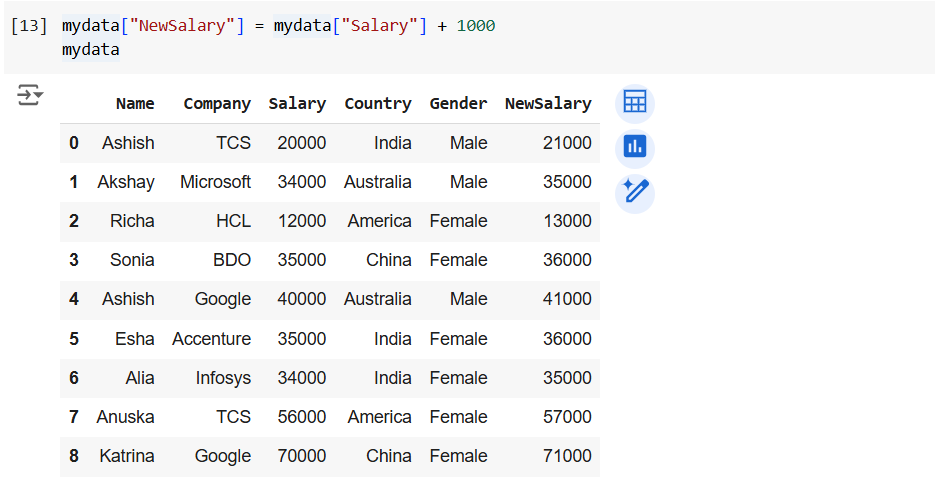
Let’s create another column named “First Language”, which has the value “English” for all the rows.
Python
mydata["First Language"] = "English" mydata
The output of the above code is shown below:
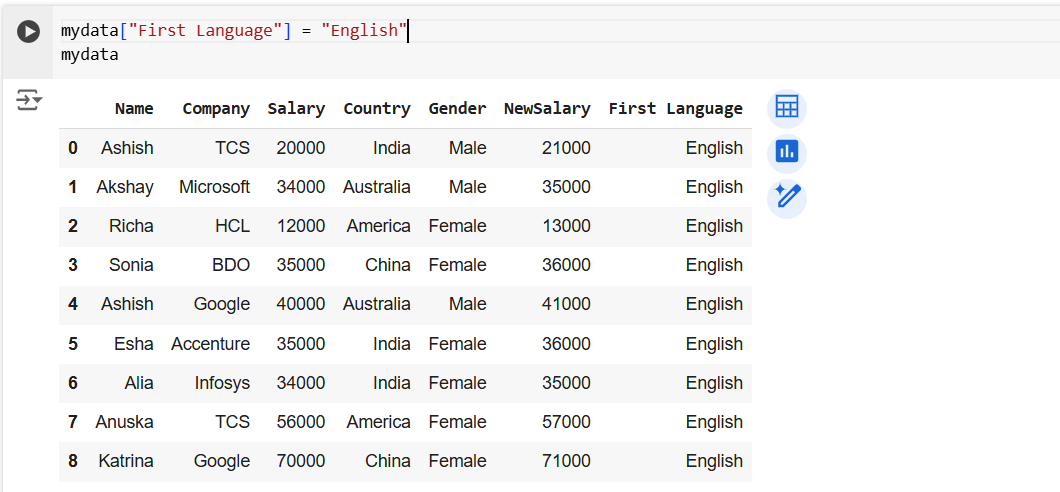